The new ContentUnavailableView() in SwiftUI
Apple's new ContentUnavailableView() is a handy abstraction for a common UI pattern.
It's things like this that make writing apps with SwiftUI so damn enjoyable. With iOS 17, Apple has introduced a new, standardized view for instances when our content is unavailable, like when the user no longer has network access, for example. It's called ContentUnavailableView()
and it makes building these common UI patterns painless, while maintaining iOS interface consistency.
How it works
ContentUnavailableView() with an SF Symbol
I suspect this will be one of the most common ways we see developers using this view, and that includes inside Apple. You provide a title
string, an SF Symbol name (systemImage
), and a Text()
view as a description
, and you get this rather pleasing UI with very little effort:
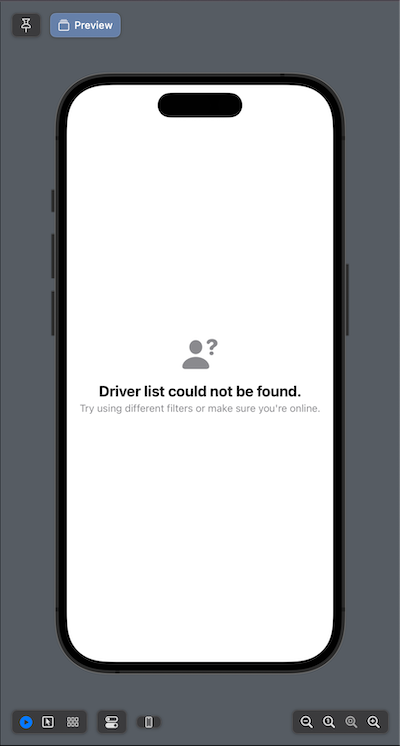
struct Driver: Hashable {
var name: String
}
struct ContentView: View {
@State private var drivers: [Driver] = []
var body: some View {
List(drivers, id: \.self) { driver in
Text(driver.name)
}
.background {
if drivers.isEmpty {
ContentUnavailableView(
"Driver list could not be found.",
systemImage: "person.fill.questionmark",
description: Text(
"Try using different filters or make sure you're online."
)
)
}
}
}
}
ContentUnavailableView() with any image
You can also use your own images with ContentUnavailableView()
. Here, image
is just the name of the image asset you wish to reference:
ContentUnavailableView("Ooops...", image: "Eli", description: Text("I couldn't find what you were looking for."))
You'll get something like this:
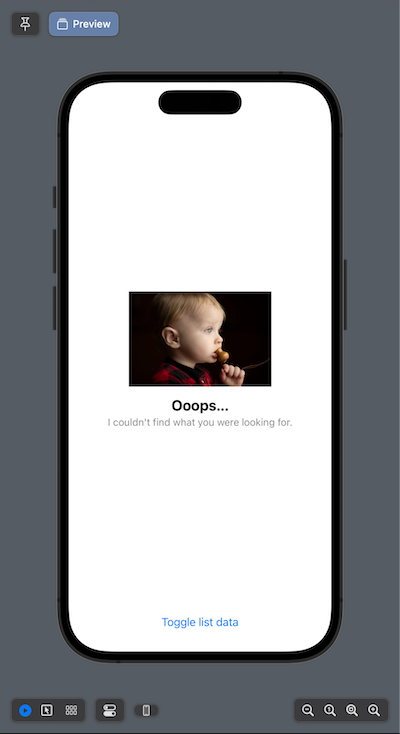
Using Apple's Built-In variants
Apple is even providing a standardized view for search interfaces. The documentation mentions:
The system provides defaultContentUnavailableView
s that you can use in specific situations. The example below illustrates the usage of thesearch
view:
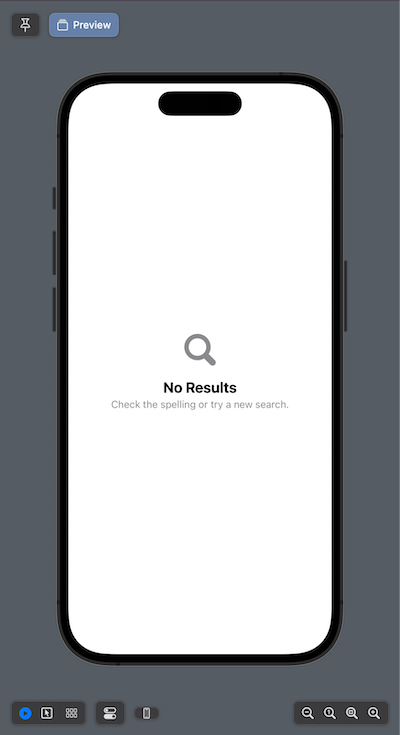
As of this writing, .search
is the only variant I see in Beta 1, but perhaps that will change in the future. In any case, it's convenient enough. You can use it like this:
ContentUnavailableView.search
All of these examples look great on light and dark modes, different accessibility settings, and in landscape orientation. Apple seems to be adding more and more of these standard views inside each release of SwiftUI and I am here for it. See also the new SubscriptionStoreView()
, which as of Beta 1, wasn't working for me in Xcode 15.